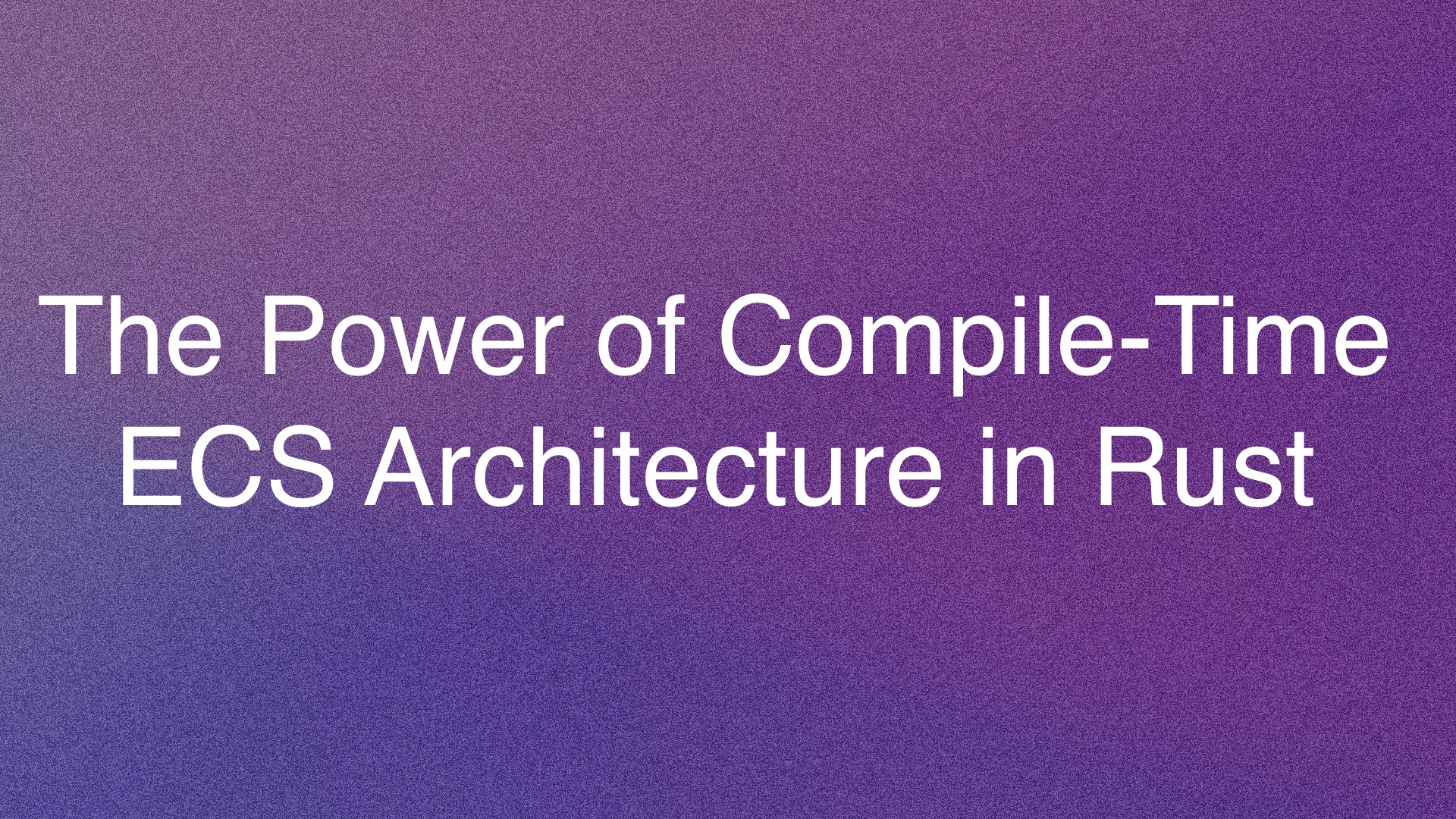
The Power of Compile-Time ECS Architecture in Rust
- Introduction
- Why Compile-Time ECS?
- Real-World Example: Robotics Controller
- Component Traits and Archetypes
- Composing Systems
- Compile-Time Initialization
- Performance Implications
- Comparing Runtime vs. Compile-Time ECS
- Conclusion
- Further Reading
Introduction
Entity-Component Systems (ECS) have become a dominant architectural pattern in game development
and simulation software, prized for their flexibility and performance.
But what if we take ECS to the next level by moving it from runtime to compile time?
This article explores how Rust’s powerful type system and const generics
can create a fully static ECS architecture,
potentially offering significant performance improvements and deterministic behavior for specialized applications.
Why Compile-Time ECS?
Traditional ECS architectures shine when flexibility is paramount—when entities can be created, destroyed, or modified at runtime. However, in domains like embedded controllers, industrial systems, or WebAssembly applications, you often know with certainty:
- The exact number of entities in your system
- The complete component configuration for each entity
- The precise execution logic for all systems
In these scenarios, a compile-time ECS can eliminate significant overhead:
- Zero dynamic allocation: All memory requirements are known and allocated at compile time
- No runtime type checks: Component access is statically verified by the type system
- No registry lookups: Direct access to components without indirection
- Deterministic memory layout: Perfect cache locality and predictable access patterns
- Compile-time validation: Impossible states become unrepresentable.
Let’s implement a robotic arm controller to demonstrate this approach in practice.
Real-World Example: Robotics Controller
Consider a factory automation system with eight robotic arms. Each arm has:
- A unique identifier (ID)
- A fixed physical location in the factory
- A temperature sensor for monitoring
- A state machine controlling operation (Idle, Active, Error)
We’ll build a tick-based controller that monitors temperature readings and transitions states based on defined thresholds—a perfect use case for a compile-time ECS approach.
Modeling the System
trait Component {}
#[derive(Debug, Clone, Copy)]
struct Location {
x: f32,
y: f32,
}
impl Component for Location {}
#[derive(Debug, Clone, Copy)]
struct TemperatureSensor {
temp_celsius: f32,
}
impl Component for TemperatureSensor {}
#[derive(Debug, Clone, Copy, PartialEq)]
enum State {
Idle,
Active,
Error,
}
impl Component for State {}
#[derive(Debug, Clone)]
struct RoboticArm<const ID: usize> {
location: Location,
sensor: TemperatureSensor,
state: State,
}
impl<const ID: usize> Component for RoboticArm<ID> {}
What makes this different from traditional ECS?
Notice how the ID
is part of the RoboticArm's
type signature through const generics.
This means each arm is a distinct type at compile time, allowing the compiler to reason about them individually.
Running the System
impl<const ID: usize> RoboticArm<ID> {
fn tick(&mut self) {
// Simulate temperature changes based on ID
self.sensor.temp_celsius += (ID as f32) * 0.3 - 0.5;
// State transition logic
self.state = match (self.state, self.sensor.temp_celsius) {
(_, t) if t > 80.0 => State::Error, // Overheating, go to error
(State::Idle, t) if t > 40.0 => State::Active, // Warm enough, activate
(State::Active, t) if t < 30.0 => State::Idle, // Cooled down, idle
(s, _) => s, // Maintain current state
};
}
const fn new() -> Self {
Self {
location: Location {
x: ID as f32,
y: (ID / 4) as f32,
},
sensor: TemperatureSensor {
temp_celsius: 30.0 + (ID as f32),
},
state: State::Idle,
}
}
}
fn print_arm<const ID: usize>(arm: &RoboticArm<ID>) {
println!(
"[ID {}] Loc=({:.1}, {:.1}) Temp={:.1} State={:?}",
ID, arm.location.x, arm.location.y, arm.sensor.temp_celsius, arm.state
);
}
fn main() {
println!("=== ROBOTIC ARM SIMULATION ===");
// Create and run arms individually since they are different types
let mut arm0 = RoboticArm::<0>::new();
let mut arm1 = RoboticArm::<1>::new();
let mut arm2 = RoboticArm::<2>::new();
let mut arm3 = RoboticArm::<3>::new();
let mut arm4 = RoboticArm::<4>::new();
let mut arm5 = RoboticArm::<5>::new();
let mut arm6 = RoboticArm::<6>::new();
let mut arm7 = RoboticArm::<7>::new();
// Simulation loop
for i in 0..5 {
println!("\n=== TICK {} ===", i);
// Update each arm individually
arm0.tick();
arm1.tick();
arm2.tick();
arm3.tick();
arm4.tick();
arm5.tick();
arm6.tick();
arm7.tick();
// Print each arm
print_arm(&arm0);
print_arm(&arm1);
print_arm(&arm2);
print_arm(&arm3);
print_arm(&arm4);
print_arm(&arm5);
print_arm(&arm6);
print_arm(&arm7);
}
}
Unit Testing ECS State Logic
With compile-time ECS, unit tests become more straightforward and type-safe:
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_transitions_to_active() {
let mut arm = RoboticArm::<99> {
location: Location { x: 0.0, y: 0.0 },
sensor: TemperatureSensor { temp_celsius: 45.0 },
state: State::Idle,
};
arm.tick();
assert_eq!(arm.state, State::Active);
}
#[test]
fn test_transitions_to_error() {
let mut arm = RoboticArm::<88> {
location: Location { x: 0.0, y: 0.0 },
sensor: TemperatureSensor { temp_celsius: 85.0 },
state: State::Active,
};
arm.tick();
assert_eq!(arm.state, State::Error);
}
}
Note how we can create test entities with arbitrary IDs that don’t even exist in our main simulation, yet the type system ensures they behave consistently with our defined logic.
Component Traits and Archetypes
The real power of compile-time ECS comes from generalizing this approach to support diverse entity types. We can create a more flexible system using traits:
// Define a general Entity trait
trait Entity {
fn update(&mut self);
fn render(&self);
}
// Allow implementation for any RoboticArm regardless of ID
impl<const ID: usize> Entity for RoboticArm<ID> {
fn update(&mut self) {
self.tick();
}
fn render(&self) {
print_arm(self);
}
}
// Create a compile-time entity collection
struct EntitySystem<E: Entity, const N: usize> {
entities: [E; N],
}
impl<E: Entity, const N: usize> EntitySystem<E, N> {
fn update_all(&mut self) {
for entity in &mut self.entities {
entity.update();
}
}
fn render_all(&self) {
for entity in &self.entities {
entity.render();
}
}
}
This pattern allows us to build type-safe entity collections with compile-time guarantees.
Composing Systems
We can build reusable systems as traits that operate on components:
trait System<T> {
fn run(component: &mut T);
}
// A system that updates state based on temperature
struct TemperatureMonitorSystem;
impl<const ID: usize> System<RoboticArm<ID>> for TemperatureMonitorSystem {
fn run(arm: &mut RoboticArm<ID>) {
// Monitor temperature and transition state if needed
if arm.sensor.temp_celsius > 80.0 {
arm.state = State::Error;
}
}
}
// A system that simulates cooling when in error state
struct CoolingSystem;
impl<const ID: usize> System<RoboticArm<ID>> for CoolingSystem {
fn run(arm: &mut RoboticArm<ID>) {
if arm.state == State::Error {
arm.sensor.temp_celsius -= 5.0;
if arm.sensor.temp_celsius < 60.0 {
arm.state = State::Idle;
}
}
}
}
With this approach, we can compose multiple systems that operate on our entities in a predictable, type-safe manner.
Compile-Time Initialization
One of the most powerful features of this approach is the ability to initialize entities at compile time:
const ARM0: RoboticArm<0> = RoboticArm::new();
const ARM1: RoboticArm<1> = RoboticArm::new();
// This won't work with format! in const contexts yet
// But we can demonstrate the concept with direct string literals
const FACTORY_SETUP: [&str; 2] = [
"Arm 0 at position (0, 0)", // Hardcoded for demonstration
"Arm 1 at position (1, 0)", // Hardcoded for demonstration
];
fn print_factory_setup() {
println!("Arm 0 at position ({}, {})", ARM0.location.x, ARM0.location.y);
println!("Arm 1 at position ({}, {})", ARM1.location.x, ARM1.location.y);
}
This allows for static registration, zero dynamic allocations, and predictable memory layout—ideal for embedded systems and performance-critical applications.
Performance Implications
The performance benefits of compile-time ECS are significant:
- Monomorphization: The Rust compiler generates specialized code for each entity type
- Inlining: Function calls can be inlined across entity boundaries
- Dead code elimination: Unused components or systems are removed entirely
- SIMD optimizations: The compiler can vectorize operations across homogeneous component arrays
- Memory locality: Components can be laid out optimally in memory
These optimizations can result in performance improvements of several orders of magnitude compared to traditional dynamic ECS implementations for applications with fixed entity counts and predictable behavior.
Comparing Runtime vs. Compile-Time ECS
Feature | Runtime ECS | Compile-Time ECS |
---|---|---|
Flexibility | Dynamic entity creation/deletion | Fixed entity set |
Type safety | Runtime checks | Compile-time verification |
Performance | Good (with optimization) | Exceptional (fully optimized) |
Memory use | Dynamic allocation | Static allocation |
Compile time | Fast | Potentially slower |
Code size | Can be larger | Highly optimized |
Development | More flexible | More constraints |
The key insight: Choose compile-time ECS when your entity structure is known in advance and performance/determinism is critical.
Conclusion
By shifting ECS logic to compile-time, we can unlock unprecedented levels of performance and determinism for systems with fixed entity topologies. While not suitable for every application, particularly those requiring dynamic entity, creation—compile-time ECS represents an exciting frontier for performance-critical domains like embedded systems, WebAssembly modules, and specialized game engines.
The most powerful ECS architecture might not be the most flexible one, but rather the one that leverages the compiler’s knowledge to eliminate runtime overhead entirely. In domains where topology and entity roles are fixed, compile-time ECS can dramatically outperform traditional approaches in both speed and reliability. Perhaps the fastest game engine isn’t dynamic at all—it’s type-safe, predictable, and entirely static.