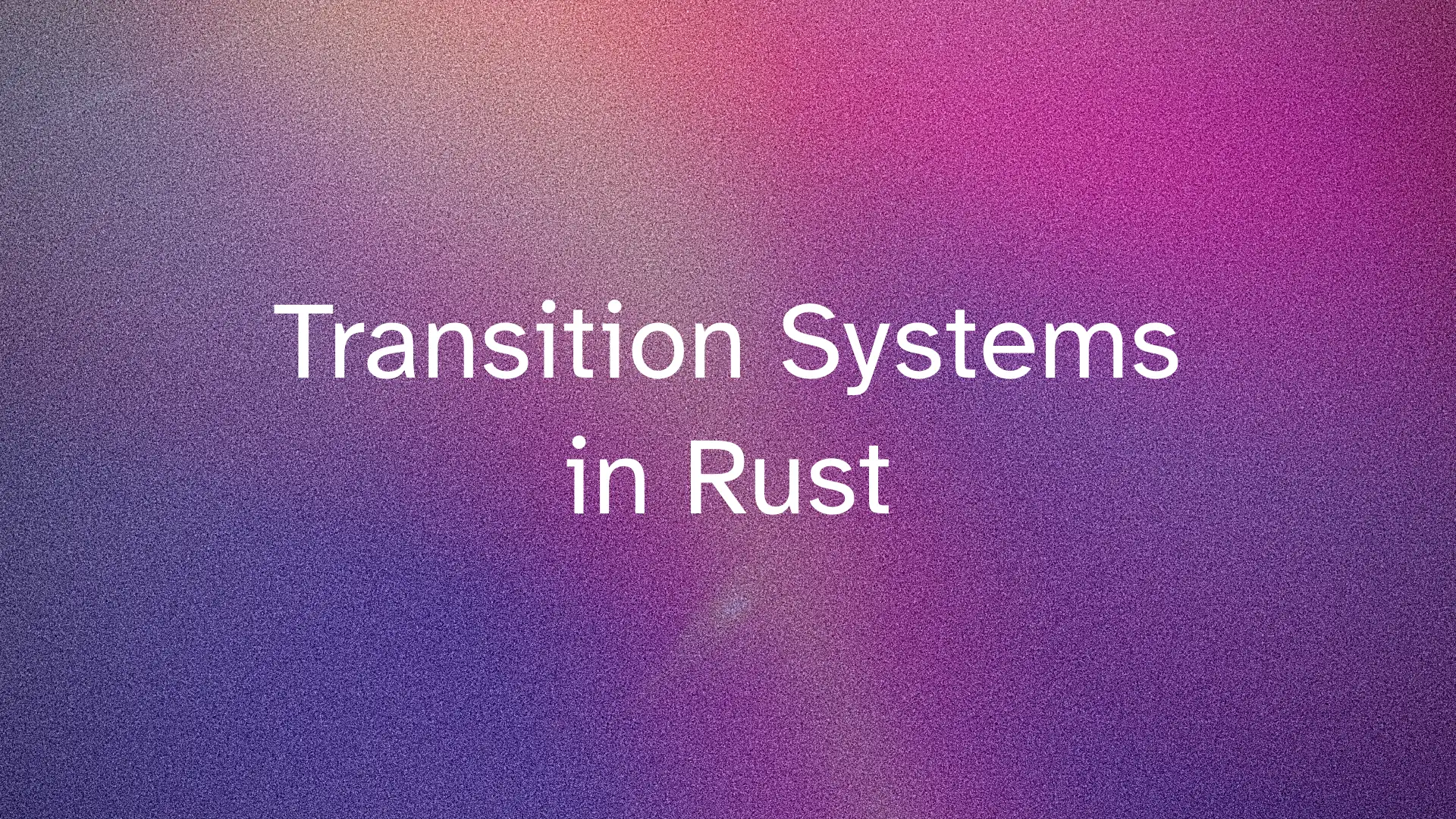
Transition Systems in Rust
- Introduction
- Implementation
- Practical Examples
- Advanced Topics
- đź§ Design Considerations and Tradeoffs
- Practical Optimization Tips
- 📊 Comparison with Existing Libraries
- đź”— Resources and Further Reading
- đź’ Final Thoughts
Introduction
Have you ever struggled with managing complex state in your applications? Whether it’s tracking a user’s journey through a checkout process, managing the lifecycle of a document, or controlling a physical device, state management is a fundamental challenge in software development.
In this post, we’ll explore how to implement robust and type-safe transition systems in Rust, leveraging the language’s powerful type system and ownership model to create maintainable state machines that prevent invalid state transitions at compile time.
đź§© What Are Transition Systems?
A transition system is a mathematical model used to describe the behavior of systems that change state in response to inputs or events. At its core, a transition system consists of:
- States: The possible configurations of the system
- Transitions: Rules that define how the system can move from one state to another
- Actions/Events: Triggers that cause state transitions
Event A Event B Event C
------ ------ ------
| | | | | |
+-------+ +-------+ +-------+ +-------+
| | | | | | | |
| State |----->| State |----->| State |----->| State |
| A | | B | | C | | D |
| | | | | | | |
+-------+ +-------+ +-------+ +-------+
^ |
| |
+-----------------------------+
Event D
Before diving into complex implementations, let’s start with a familiar example that everyone can understand.
🚦 A Simple Example: Traffic Light
Consider a traffic light system with three states: Red, Yellow, and Green. The light transitions from one state to another based on timer events:
Timer Timer Timer
----- ----- -----
| | | | | |
+-------+ +-------+ +-------+ +-------+
| | | | | | | |
| Red |----->| Green |----->|Yellow |----->| Red |
| | | | | | | |
+-------+ +-------+ +-------+ +-------+
^ |
| |
+-------------------------------------------+
This system has well-defined rules:
- Red transitions to Green after a timer event
- Green transitions to Yellow after a timer event
- Yellow transitions to Red after a timer event
We might also want to handle special cases, like an emergency override that can transition any state directly to Red.
Let’s see how we would represent this in Rust code:
// Define our states
enum TrafficLightState {
Red,
Yellow,
Green,
}
// Define events that can trigger transitions
enum TrafficLightEvent {
Timer,
Emergency,
}
// A simple implementation
impl TrafficLightState {
fn next(self, event: TrafficLightEvent) -> TrafficLightState {
match (self, event) {
// Normal cycle
(TrafficLightState::Red, TrafficLightEvent::Timer) => TrafficLightState::Green,
(TrafficLightState::Green, TrafficLightEvent::Timer) => TrafficLightState::Yellow,
(TrafficLightState::Yellow, TrafficLightEvent::Timer) => TrafficLightState::Red,
// Emergency override
(_, TrafficLightEvent::Emergency) => TrafficLightState::Red,
}
}
}
// Usage
fn main() {
let mut state = TrafficLightState::Red;
println!("Initial state: {:?}", state);
// Normal cycle
state = state.next(TrafficLightEvent::Timer); // Red -> Green
println!("After timer: {:?}", state);
// Emergency
state = state.next(TrafficLightEvent::Emergency); // Green -> Red
println!("After emergency: {:?}", state);
}
This example is straightforward, but real-world state machines quickly become more complex. They might have:
- Many more states and transitions
- Guards that conditionally prevent transitions
- Side effects during transitions
- Complex state data
Let’s build a more comprehensive framework to handle these requirements.
Implementation
🏗️ Building Blocks of a Transition System
Before diving into the code, let’s identify the key components we’ll need:
- States: Represented as Rust enums to model mutually exclusive states
- Transitions: Implemented as methods or functions that transform one state into another
- Guards: Conditions that must be satisfied before a transition can occur
- Actions: Side effects that happen during transitions
Now, let’s implement a generic transition system framework in Rust.
⚙️ Core Implementation
We’ll break down our implementation into manageable components to make it easier to understand.
State and Transition Traits
First, let’s define the core traits that will form the foundation of our system:
use std::fmt::Debug;
/// Trait for types that can be used as states in a transition system.
pub trait State: Clone + Debug + PartialEq {}
/// Automatically implement State for any type that satisfies the required bounds.
impl<T: Clone + Debug + PartialEq> State for T {}
/// Trait for types that define transitions between states.
pub trait Transition<S: State> {
/// The event type that triggers this transition.
type Event;
/// The error type that may be returned if a transition fails.
type Error;
/// Apply a transition based on the current state and an event.
/// Returns either the new state or an error if the transition is invalid.
fn apply(&self, state: &S, event: Self::Event) -> Result<S, Self::Error>;
/// Check if a transition is valid for the current state and event
/// without actually performing the transition.
fn is_valid(&self, state: &S, event: &Self::Event) -> bool;
}
/// Possible errors during state transitions.
#[derive(Debug, Clone, PartialEq)]
pub enum TransitionError {
/// The transition is not allowed from the current state.
InvalidTransition,
/// A guard condition prevented the transition.
GuardFailed(String),
/// A custom error occurred during the transition.
Custom(String),
}
These traits define:
- What makes a type usable as a state (cloneable, debuggable, comparable)
- How transitions operate on states (apply, check validity)
- Standard error types for transitions
TypedTransition Implementation
Next, let’s implement a concrete transition type that enforces type safety:
use std::marker::PhantomData;
/// A typed transition that can only be applied to specific source and target states.
pub struct TypedTransition<S, E, Src, Tgt, F>
where
S: State,
Src: 'static,
Tgt: 'static,
F: Fn(&S, E) -> Result<S, TransitionError>,
{
transition_fn: F,
_source_state: PhantomData<Src>,
_target_state: PhantomData<Tgt>,
_state: PhantomData<S>,
_event: PhantomData<E>,
}
impl<S, E, Src, Tgt, F> TypedTransition<S, E, Src, Tgt, F>
where
S: State,
Src: 'static,
Tgt: 'static,
F: Fn(&S, E) -> Result<S, TransitionError>,
{
/// Create a new typed transition with the provided transition function.
pub fn new(transition_fn: F) -> Self {
Self {
transition_fn,
_source_state: PhantomData,
_target_state: PhantomData,
_state: PhantomData,
_event: PhantomData,
}
}
}
impl<S, E, Src, Tgt, F> Transition<S> for TypedTransition<S, E, Src, Tgt, F>
where
S: State,
E: Clone,
Src: 'static,
Tgt: 'static,
F: Fn(&S, E) -> Result<S, TransitionError>,
{
type Event = E;
type Error = TransitionError;
fn apply(&self, state: &S, event: Self::Event) -> Result<S, Self::Error> {
(self.transition_fn)(state, event)
}
fn is_valid(&self, state: &S, event: &Self::Event) -> bool {
match (self.transition_fn)(state, event.clone()) {
Ok(_) => true,
Err(_) => false,
}
}
}
The TypedTransition
struct uses Rust’s type system to enforce constraints on transitions, using phantom types to track source and target states.
TransitionSystem
Now, let’s implement the main system that manages the current state and registered transitions:
/// A transition system that manages states and transitions.
pub struct TransitionSystem<S, E>
where
S: State,
{
current_state: S,
transitions: Vec<Box<dyn Transition<S, Event = E, Error = TransitionError>>>,
}
impl<S, E> TransitionSystem<S, E>
where
S: State,
E: Clone,
{
/// Create a new transition system with the given initial state.
pub fn new(initial_state: S) -> Self {
Self {
current_state: initial_state,
transitions: Vec::new(),
}
}
/// Register a transition in the system.
pub fn register_transition<T>(&mut self, transition: T)
where
T: Transition<S, Event = E, Error = TransitionError> + 'static,
{
self.transitions.push(Box::new(transition));
}
/// Apply an event to trigger a state transition.
pub fn apply_event(&mut self, event: E) -> Result<&S, TransitionError> {
for transition in &self.transitions {
if transition.is_valid(&self.current_state, &event) {
match transition.apply(&self.current_state, event.clone()) {
Ok(new_state) => {
self.current_state = new_state;
return Ok(&self.current_state);
}
Err(e) => return Err(e),
}
}
}
Err(TransitionError::InvalidTransition)
}
/// Get the current state of the system.
pub fn current_state(&self) -> &S {
&self.current_state
}
/// Check if a transition is possible from the current state.
pub fn can_transition(&self, event: &E) -> bool {
self.transitions.iter().any(|t| t.is_valid(&self.current_state, event))
}
/// Get all possible transitions from the current state.
pub fn possible_transitions(&self, events: &[E]) -> Vec<E>
where
E: Clone,
{
events.iter().filter(|e| self.can_transition(e)).cloned().collect()
}
}
The TransitionSystem
manages:
- The current state of the system
- A collection of registered transitions
- Application of events to trigger transitions
- Querying for possible transitions from the current state
TransitionBuilder
Finally, let’s create a builder pattern to make it easy to define transitions:
use std::cell::RefCell;
use std::rc::Rc;
/// A builder for creating typed transitions with guards and actions.
pub struct TransitionBuilder<S, E>
where
S: State,
{
source_states: Vec<S>,
target_state: Option<S>,
event: Option<E>,
guards: Vec<Box<dyn Fn(&S, &E) -> Result<(), String>>>,
actions: Vec<Box<dyn FnMut(&S, &E)>>,
}
impl<S, E> TransitionBuilder<S, E>
where
S: State,
E: Clone,
{
/// Create a new transition builder.
pub fn new() -> Self {
Self {
source_states: Vec::new(),
target_state: None,
event: None,
guards: Vec::new(),
actions: Vec::new(),
}
}
/// Set the source state for this transition.
pub fn from(mut self, state: S) -> Self {
self.source_states.push(state);
self
}
/// Set the target state for this transition.
pub fn to(mut self, state: S) -> Self {
self.target_state = Some(state);
self
}
/// Set the event that triggers this transition.
pub fn on_event(mut self, event: E) -> Self {
self.event = Some(event);
self
}
/// Add a guard condition to this transition.
pub fn guard<F>(mut self, guard_fn: F) -> Self
where
F: Fn(&S, &E) -> Result<(), String> + 'static,
{
self.guards.push(Box::new(guard_fn));
self
}
/// Add an action to be performed during this transition.
pub fn action<F>(mut self, action_fn: F) -> Self
where
F: FnMut(&S, &E) + 'static,
{
self.actions.push(Box::new(action_fn));
self
}
/// Build the transition and return a boxed Transition trait object.
pub fn build(self) -> impl Transition<S, Event = E, Error = TransitionError> + 'static
where
S: 'static,
E: 'static,
{
let source_states = self.source_states;
let target_state = self.target_state.expect("Target state must be set");
let _event_template = self.event.expect("Event must be set");
let guards = self.guards;
// We're going to use Rc<RefCell<...>> to allow mutation inside a Fn closure
let actions = Rc::new(RefCell::new(self.actions));
TypedTransition::<S, E, (), (), _>::new(move |state: &S, event: E| {
// Check if the current state is a valid source state
if !source_states.is_empty() && !source_states.iter().any(|s| s == state) {
return Err(TransitionError::InvalidTransition);
}
// Check if all guards pass
for guard in &guards {
if let Err(msg) = guard(state, &event) {
return Err(TransitionError::GuardFailed(msg));
}
}
// Execute all actions
if let Ok(mut actions_ref) = actions.try_borrow_mut() {
for action in &mut *actions_ref {
action(state, &event);
}
}
// Return the new state
Ok(target_state.clone())
})
}
}
The TransitionBuilder
provides a fluent API for creating transitions with guards and actions, making the code more readable and maintainable.
Practical Examples
Now that we have our framework in place, let’s explore some practical examples to see it in action.
🚦 The Traffic Light Controller
Let’s revisit our traffic light example with our new framework:
// Define our states and events
#[derive(Debug, Clone, PartialEq)]
enum TrafficLightState {
Red,
Yellow,
Green,
}
#[derive(Debug, Clone, PartialEq)]
enum TrafficLightEvent {
Timer,
Emergency,
}
// Create a traffic light controller
fn main() {
// Create a new transition system
let mut system = TransitionSystem::new(TrafficLightState::Red);
// Define the normal cycle transitions
let red_to_green = TransitionBuilder::new()
.from(TrafficLightState::Red)
.to(TrafficLightState::Green)
.on_event(TrafficLightEvent::Timer)
.action(|_, _| println!("Changing from Red to Green"))
.build();
let green_to_yellow = TransitionBuilder::new()
.from(TrafficLightState::Green)
.to(TrafficLightState::Yellow)
.on_event(TrafficLightEvent::Timer)
.action(|_, _| println!("Changing from Green to Yellow"))
.build();
let yellow_to_red = TransitionBuilder::new()
.from(TrafficLightState::Yellow)
.to(TrafficLightState::Red)
.on_event(TrafficLightEvent::Timer)
.action(|_, _| println!("Changing from Yellow to Red"))
.build();
// Define the emergency transition
let emergency_to_red = TransitionBuilder::new()
.from(TrafficLightState::Green)
.from(TrafficLightState::Yellow)
.to(TrafficLightState::Red)
.on_event(TrafficLightEvent::Emergency)
.action(|_, _| println!("EMERGENCY! Changing to Red"))
.build();
// Register all transitions
system.register_transition(red_to_green);
system.register_transition(green_to_yellow);
system.register_transition(yellow_to_red);
system.register_transition(emergency_to_red);
// Simulate the traffic light cycling
println!("Initial state: {:?}", system.current_state());
// Cycle through the normal sequence
system.apply_event(TrafficLightEvent::Timer).unwrap(); // Red -> Green
println!("Current state: {:?}", system.current_state());
system.apply_event(TrafficLightEvent::Timer).unwrap(); // Green -> Yellow
println!("Current state: {:?}", system.current_state());
system.apply_event(TrafficLightEvent::Timer).unwrap(); // Yellow -> Red
println!("Current state: {:?}", system.current_state());
// Simulate an emergency
system.apply_event(TrafficLightEvent::Timer).unwrap(); // Red -> Green
println!("Current state: {:?}", system.current_state());
system.apply_event(TrafficLightEvent::Emergency).unwrap(); // Green -> Red
println!("Current state: {:?}", system.current_state());
}
This example demonstrates:
- How to define states and events as enums
- How to create transitions with actions
- How to apply events to trigger transitions
Let’s move on to a more complex example.
🔄 Example: Building a Document Workflow System
Now, let’s implement a document workflow system, which is a common use case in business applications where documents move through different states of approval.
+----------------+
| |
v |
+--------+ Submit +--------+ Reject +---------+
| |--------->| |--------->| |
| Draft | | Review | | Rejected|
| | | | | |
+--------+ +--------+ +---------+
^ |
| | Approve
| v
| +-----------+ Publish +-----------+
| | |---------->| |
+--------------| Approved | | Published |
Revise | | | |
+-----------+ +-----------+
First, let’s define our states and events:
/// Document states in a workflow system
#[derive(Debug, Clone, PartialEq)]
enum DocumentState {
Draft,
Review,
Approved,
Published,
Rejected,
}
/// Events that can trigger state transitions
#[derive(Debug, Clone, PartialEq)]
enum DocumentEvent {
Submit,
Approve,
Reject,
Publish,
Revise,
}
/// Document with metadata and content
#[derive(Debug)]
struct Document {
id: String,
title: String,
content: String,
state: DocumentState,
author: String,
reviewer: Option<String>,
}
impl Document {
fn new(id: &str, title: &str, author: &str) -> Self {
Self {
id: id.to_string(),
title: title.to_string(),
content: String::new(),
state: DocumentState::Draft,
author: author.to_string(),
reviewer: None,
}
}
fn set_reviewer(&mut self, reviewer: &str) {
self.reviewer = Some(reviewer.to_string());
}
fn update_content(&mut self, content: &str) {
self.content = content.to_string();
}
}
Now, let’s create a document workflow manager:
/// The document workflow manager
struct DocumentWorkflow {
system: TransitionSystem<DocumentState, DocumentEvent>,
document: Document,
}
impl DocumentWorkflow {
fn new(document: Document) -> Self {
let mut system = TransitionSystem::new(document.state.clone());
// Define transitions
// Draft -> Review
let submit = TransitionBuilder::new()
.from(DocumentState::Draft)
.to(DocumentState::Review)
.on_event(DocumentEvent::Submit)
.guard(|_, _| {
// In a real system, we might check document length, etc.
Ok(())
})
.build();
// Review -> Approved
let approve = TransitionBuilder::new()
.from(DocumentState::Review)
.to(DocumentState::Approved)
.on_event(DocumentEvent::Approve)
.guard(|_, _| {
// In a real system, we might verify reviewer permissions
Ok(())
})
.build();
// Review -> Rejected
let reject = TransitionBuilder::new()
.from(DocumentState::Review)
.to(DocumentState::Rejected)
.on_event(DocumentEvent::Reject)
.build();
// Approved -> Published
let publish = TransitionBuilder::new()
.from(DocumentState::Approved)
.to(DocumentState::Published)
.on_event(DocumentEvent::Publish)
.build();
// Rejected -> Draft
let revise = TransitionBuilder::new()
.from(DocumentState::Rejected)
.to(DocumentState::Draft)
.on_event(DocumentEvent::Revise)
.build();
// Register all transitions
system.register_transition(submit);
system.register_transition(approve);
system.register_transition(reject);
system.register_transition(publish);
system.register_transition(revise);
Self { system, document }
}
fn apply_event(&mut self, event: DocumentEvent) -> Result<(), TransitionError> {
let new_state = self.system.apply_event(event)?;
self.document.state = new_state.clone();
println!("Document '{}' transitioned to state: {:?}", self.document.title, new_state);
Ok(())
}
fn current_state(&self) -> &DocumentState {
self.system.current_state()
}
fn possible_transitions(&self) -> Vec<DocumentEvent> {
let all_events = vec![
DocumentEvent::Submit,
DocumentEvent::Approve,
DocumentEvent::Reject,
DocumentEvent::Publish,
DocumentEvent::Revise,
];
self.system.possible_transitions(&all_events)
}
}
Let’s use our document workflow:
fn main() {
let doc = Document::new("DOC-001", "Quarterly Report", "Alice");
let mut workflow = DocumentWorkflow::new(doc);
println!("Initial state: {:?}", workflow.current_state());
println!("Possible transitions: {:?}", workflow.possible_transitions());
// Walk through the workflow
println!("\nSubmitting document for review...");
workflow.apply_event(DocumentEvent::Submit).expect("Failed to submit");
println!("Possible transitions: {:?}", workflow.possible_transitions());
println!("\nApproving document...");
workflow.apply_event(DocumentEvent::Approve).expect("Failed to approve");
println!("Possible transitions: {:?}", workflow.possible_transitions());
println!("\nPublishing document...");
workflow.apply_event(DocumentEvent::Publish).expect("Failed to publish");
println!("Possible transitions: {:?}", workflow.possible_transitions());
// This should fail - can't revise a published document
println!("\nAttempting to revise a published document...");
match workflow.apply_event(DocumentEvent::Revise) {
Ok(_) => println!("Successfully revised (unexpected)"),
Err(e) => println!("Failed as expected: {:?}", e),
}
}
🖨️ Expected Output
Initial state: Draft
Possible transitions: [Submit]
Submitting document for review...
Document 'Quarterly Report' transitioned to state: Review
Possible transitions: [Approve, Reject]
Approving document...
Document 'Quarterly Report' transitioned to state: Approved
Possible transitions: [Publish]
Publishing document...
Document 'Quarterly Report' transitioned to state: Published
Possible transitions: []
Attempting to revise a published document...
Failed as expected: InvalidTransition
This example demonstrates:
- Creating a domain-specific workflow with states and events
- Using guards to conditionally allow transitions
- Querying for possible transitions from the current state
- Handling invalid transitions gracefully
Advanced Topics
đź§µ Concurrency and Thread Safety
One of Rust’s strengths is its ability to provide thread-safety guarantees at compile time. Let’s explore how to make our transition system thread-safe:
use std::sync::{Arc, Mutex, RwLock};
/// A thread-safe transition system
pub struct ThreadSafeTransitionSystem<S, E>
where
S: State + Send + Sync,
E: Clone + Send + Sync,
{
// Using RwLock for the state allows multiple readers but exclusive writers
current_state: RwLock<S>,
// Using Arc for shared ownership of transitions
transitions: Arc<Vec<Box<dyn Transition<S, Event = E, Error = TransitionError> + Send + Sync>>>,
}
impl<S, E> ThreadSafeTransitionSystem<S, E>
where
S: State + Send + Sync + 'static,
E: Clone + Send + Sync + 'static,
{
pub fn new(initial_state: S) -> Self {
Self {
current_state: RwLock::new(initial_state),
transitions: Arc::new(Vec::new()),
}
}
pub fn register_transition<T>(&mut self, transition: T)
where
T: Transition<S, Event = E, Error = TransitionError> + Send + Sync + 'static,
{
let mut transitions = Arc::get_mut(&mut self.transitions)
.expect("Cannot modify transitions after sharing");
transitions.push(Box::new(transition));
}
pub fn apply_event(&self, event: E) -> Result<S, TransitionError> {
// Get a read lock to check if transition is valid
let current_state = self.current_state.read().unwrap();
// Find a valid transition
let mut valid_transition = None;
for transition in self.transitions.iter() {
if transition.is_valid(¤t_state, &event) {
valid_transition = Some(transition);
break;
}
}
// Drop the read lock
drop(current_state);
if let Some(transition) = valid_transition {
// Get exclusive write access to state
let mut state_guard = self.current_state.write().unwrap();
// Apply the transition
match transition.apply(&state_guard, event) {
Ok(new_state) => {
// Update state
*state_guard = new_state.clone();
Ok(new_state)
}
Err(e) => Err(e),
}
} else {
Err(TransitionError::InvalidTransition)
}
}
// Other methods omitted for brevity
}
Using this thread-safe implementation:
fn main() {
let system = Arc::new(ThreadSafeTransitionSystem::new(TrafficLight::Red));
// Create multiple threads that apply events
let mut handles = vec![];
for i in 0..10 {
let system_clone = system.clone();
let handle = std::thread::spawn(move || {
// Each thread applies a different pattern of events
match i % 3 {
0 => system_clone.apply_event(TrafficEvent::Timer),
1 => system_clone.apply_event(TrafficEvent::Emergency),
_ => system_clone.apply_event(TrafficEvent::Reset),
}
});
handles.push(handle);
}
// Wait for all threads to complete
for handle in handles {
handle.join().unwrap();
}
// Final state will depend on the order of thread execution
println!("Final state: {:?}", system.current_state());
}
Key points:
RwLock
allows multiple readers but exclusive writersArc
enables shared ownership across threadsSend + Sync
trait bounds ensure thread safety- Two-phase locking (read then write) reduces contention
đź’ľ Persistence and Serialization
In many applications, you need to persist state and reload it later. Let’s add serialization support:
use serde::{Serialize, Deserialize};
#[derive(Debug, Clone, PartialEq, Serialize, Deserialize)]
enum DocumentState {
Draft,
Review,
Approved,
Published,
Rejected,
}
#[derive(Debug, Clone, PartialEq, Serialize, Deserialize)]
struct DocumentWithHistory {
document: Document,
state_history: Vec<(DocumentState, String)>, // (state, timestamp)
}
impl DocumentWorkflow {
// Save the current state to a file
fn save_state(&self, path: &str) -> std::io::Result<()> {
let serialized = serde_json::to_string_pretty(&self.document)?;
std::fs::write(path, serialized)?;
Ok(())
}
// Load state from a file
fn load_state(path: &str) -> std::io::Result<Self> {
let data = std::fs::read_to_string(path)?;
let document: Document = serde_json::from_str(&data)?;
Ok(Self::new(document))
}
// Record state transitions for audit
fn apply_event_with_history(&mut self, event: DocumentEvent) -> Result<(), TransitionError> {
// Store old state for comparison
let old_state = self.document.state.clone();
// Apply the transition
self.apply_event(event)?;
// If state changed, record it in history
if old_state != self.document.state {
let timestamp = chrono::Utc::now().to_rfc3339();
println!("State transition at {}: {:?} -> {:?}",
timestamp, old_state, self.document.state);
// In a real system, this would be persisted
}
Ok(())
}
}
This implementation:
- Uses Serde for serialization
- Records state transition history
- Provides load/save functionality
🔌 Integration with Web Services and GUIs
Let’s see how our transition system can integrate with a web service:
// Using actix-web as an example
use actix_web::{web, App, HttpResponse, HttpServer, Responder};
// Shared state for our web service
struct AppState {
workflow: Mutex<DocumentWorkflow>,
}
// API endpoints
async fn get_document_state(data: web::Data<AppState>) -> impl Responder {
let workflow = data.workflow.lock().unwrap();
let state = workflow.current_state().clone();
HttpResponse::Ok().json(state)
}
async fn apply_document_event(
event: web::Json<DocumentEvent>,
data: web::Data<AppState>
) -> impl Responder {
let mut workflow = data.workflow.lock().unwrap();
match workflow.apply_event(event.0) {
Ok(_) => HttpResponse::Ok().body("Event applied successfully"),
Err(e) => HttpResponse::BadRequest().body(format!("Failed to apply event: {:?}", e)),
}
}
// Main function for the web service
#[actix_web::main]
async fn main() -> std::io::Result<()> {
// Create document and workflow
let document = Document::new("DOC-001", "User Guide", "Alice");
let workflow = DocumentWorkflow::new(document);
// Create shared state
let app_state = web::Data::new(AppState {
workflow: Mutex::new(workflow),
});
// Start web server
HttpServer::new(move || {
App::new()
.app_data(app_state.clone())
.route("/state", web::get().to(get_document_state))
.route("/event", web::post().to(apply_document_event))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
For a GUI application using a framework like iced
:
use iced::{button, Button, Column, Element, Sandbox, Settings, Text};
struct DocumentApp {
workflow: DocumentWorkflow,
submit_button: button::State,
approve_button: button::State,
reject_button: button::State,
publish_button: button::State,
revise_button: button::State,
}
#[derive(Debug, Clone)]
enum Message {
Submit,
Approve,
Reject,
Publish,
Revise,
}
impl Sandbox for DocumentApp {
type Message = Message;
fn new() -> Self {
// Create document and workflow
let document = Document::new("DOC-001", "User Guide", "Alice");
Self {
workflow: DocumentWorkflow::new(document),
submit_button: button::State::new(),
approve_button: button::State::new(),
reject_button: button::State::new(),
publish_button: button::State::new(),
revise_button: button::State::new(),
}
}
fn title(&self) -> String {
format!("Document Workflow - {:?}", self.workflow.current_state())
}
fn update(&mut self, message: Message) {
// Map GUI message to document event
let event = match message {
Message::Submit => DocumentEvent::Submit,
Message::Approve => DocumentEvent::Approve,
Message::Reject => DocumentEvent::Reject,
Message::Publish => DocumentEvent::Publish,
Message::Revise => DocumentEvent::Revise,
};
// Apply the event to the workflow
let _ = self.workflow.apply_event(event);
}
fn view(&mut self) -> Element<Message> {
// Get possible transitions
let possible_transitions = self.workflow.possible_transitions();
// Create buttons for each possible transition
let submit_button = Button::new(&mut self.submit_button, Text::new("Submit"))
.on_press_maybe(
if possible_transitions.contains(&DocumentEvent::Submit) {
Some(Message::Submit)
} else {
None
}
);
// Create buttons for other transitions (omitted for brevity)
// Create layout
Column::new()
.push(Text::new(format!("State: {:?}", self.workflow.current_state())))
.push(submit_button)
// Add other buttons here
.into()
}
}
These examples show how to integrate our transition system with:
- A REST API using actix-web
- A desktop GUI application using iced
đź§ Design Considerations and Tradeoffs
When implementing transition systems, several design considerations and tradeoffs come into play. Let’s explore some key aspects to consider:
State Representation
Enum vs. Trait:
- Enum Approach: As demonstrated in our examples, using enums for state representation provides compile-time exhaustiveness checking and clear state boundaries.
enum DocumentState { Draft, Review, Approved, Published, Rejected }
- Trait Approach: Alternatively, states could be represented as types implementing a common trait, enabling more flexible state-specific data and behavior.
trait State { fn name(&self) -> &'static str; fn allowed_transitions(&self) -> Vec<&'static str>; } struct DraftState; struct ReviewState { reviewer: String }
Tradeoff: Enums are simpler but less extensible; traits allow more extensibility but require more boilerplate.
Transition Storage
Vector vs. HashMap:
- Our implementation uses a
Vec<Box<dyn Transition>>
to store transitions, which works well for small to medium numbers of transitions. - For systems with many states and transitions, a HashMap-based approach might be more efficient:
HashMap<(StateId, EventType), Box<dyn Transition>>
Tradeoff: Sequential searching (Vec) is simpler but slower for many transitions; HashMap lookup is faster but requires more complex key management.
Error Handling
Result vs. Option:
- Our implementation uses
Result<S, TransitionError>
to provide rich error information. - A simpler approach might use
Option<S>
for cases where detailed error reporting isn’t needed.
Tradeoff: Result provides more context but requires more error handling code; Option is simpler but less informative.
Recovery Strategies:
// Various approaches to error recovery:
// 1. Return to last valid state
let backup_state = current_state.clone();
if let Err(_) = system.apply_event(event) {
system.force_state(backup_state);
}
// 2. Transaction-like approach with multiple events
fn apply_transaction(&mut self, events: Vec<Event>) -> Result<(), TransitionError> {
let original_state = self.current_state.clone();
for event in events {
if let Err(e) = self.apply_event(event) {
// Rollback to original state on any failure
self.current_state = original_state;
return Err(e);
}
}
Ok(())
}
// 3. Retry with fallback events
fn apply_with_fallback(&mut self, primary: Event, fallback: Event) -> Result<(), TransitionError> {
match self.apply_event(primary) {
Ok(_) => Ok(()),
Err(_) => self.apply_event(fallback),
}
}
Type Safety vs. Flexibility
Static vs. Dynamic Checking:
- Our
TypedTransition
provides compile-time guarantees about valid transitions. - A more dynamic approach might check validity at runtime, enabling transitions to be defined from configuration.
Tradeoff: Static checking catches errors earlier but is less flexible; dynamic checking allows runtime configuration but pushes errors to runtime.
Composability
Nested State Machines:
- For complex systems, consider supporting hierarchical state machines:
enum MainState { Idle, Active(ActiveSubState), Error, } enum ActiveSubState { Initializing, Running, Pausing, }
Tradeoff: Hierarchical states model complex systems better but increase implementation complexity.
Practical Optimization Tips
While raw performance is rarely the bottleneck in transition systems, here are some practical optimizations:
-
Avoid Cloning Large States: For states containing large data, consider using references or Arc/Rc.
struct Document { content: Arc<String>, // Avoid cloning large content // ... }
-
Lazy Guard Evaluation: Evaluate expensive guards only when necessary.
.guard(|state, event| { // Check simple conditions first if !basic_condition(state) { return Err("Basic condition failed".to_string()); } // Only then do expensive check expensive_validation(state, event) })
-
Preallocate Transitions: For systems with a fixed set of states and transitions, preallocate collections.
-
Consider Event Batching: For high-throughput systems, batch process events to reduce overhead.
These considerations will help you design transition systems that balance correctness, maintainability, and performance for your specific use case.
📊 Comparison with Existing Libraries
Several Rust libraries already exist for implementing state machines and transition systems. Let’s compare our approach with some popular options:
Library | Approach | Pros | Cons |
---|---|---|---|
Our Implementation | Type-safe transition system with builders | Custom fit, educational, flexible | Requires more code than using an existing library |
stateful | Procedural macros for state machines | Minimal boilerplate, elegant syntax | Less explicit about transitions, macro-based |
state_machine_future | Async state machines with futures | Great for async workflows | Limited to futures-based applications |
smlang | Macro-based DSL for state machines | Concise syntax, visualization | Steeper learning curve for the DSL |
When to use each:
- Our Implementation: When you need full control, want to understand the internals, or have specific requirements not met by existing libraries.
- stateful: For simpler state machines with standard patterns and minimal boilerplate.
- state_machine_future: When your state machine is part of an asynchronous workflow.
- smlang: When you want a domain-specific language for state machines with visualization capabilities.
Example using stateful
:
use stateful::{AsStateful, Stateful, Container};
#[derive(Debug, PartialEq, Copy, Clone)]
enum TrafficLightState {
Red,
Yellow,
Green,
}
#[derive(Debug, PartialEq, Copy, Clone)]
enum TrafficLightEvent {
Timer,
Emergency,
}
#[derive(Debug, AsStateful)]
struct TrafficLightStateful {
#[stateful(state)]
state: TrafficLightState,
}
impl Stateful for TrafficLightStateful {
type State = TrafficLightState;
type Trigger = TrafficLightEvent;
fn transition(&mut self, trigger: &Self::Trigger) -> Result<(), String> {
use TrafficLightState::*;
use TrafficLightEvent::*;
let next_state = match (self.state, trigger) {
(Red, Timer) => Green,
(Green, Timer) => Yellow,
(Yellow, Timer) => Red,
(_, Emergency) => Red,
};
self.state = next_state;
Ok(())
}
}
đź”— Resources and Further Reading
For those interested in learning more about state machines and transition systems, here are some valuable resources:
-
Books and Papers
- Formal Methods: State Machines by Leslie Lamport
- Finite State Machines and Regular Expressions - Stanford CS143
- Statecharts: A Visual Formalism for Complex Systems by David Harel
-
Rust-Specific Resources
- Finite State Machines in Rust by Ana Hobden
- Pretty State Machine Patterns in Rust by Yoshua Wuyts
- State Machines in Rust - Documentation for state_machine_future
- The Typestate Pattern in Rust by Cliff L. Biffle
-
Tools
- SMLANG Rust Crate - State Machine Language for Rust
- PlantUML State Diagram Syntax - For visualizing state machines
- Statecharts.dev - Learning resource for statecharts
- Mermaid.js State Diagrams - Lightweight diagramming
-
Design Patterns
- State Pattern - Object-oriented approach to state machines
- The State Machine Design Pattern from Game Programming Patterns
đź’ Final Thoughts
Transition systems provide a powerful paradigm for modeling state-based behavior in software applications. By leveraging Rust’s type system, we’ve created a framework that:
- Provides Compile-Time Safety: Invalid state transitions are caught at compile time rather than runtime.
- Is Generic and Reusable: The framework can be used for various domains from UI to backend workflows.
- Maintains High Performance: The zero-cost abstractions in Rust ensure our transition system adds minimal overhead.
- Offers Clear Intent: The DSL-like builder pattern makes the transitions easy to understand and maintain.
In real-world applications, you might extend this framework with:
- Persistence: Saving and loading state from databases
- Distributed Transitions: Coordinating transitions across multiple nodes
- Visualization: Generating diagrams from the transition definitions
- Event Sourcing: Recording the history of transitions for auditing or replay
This approach to state management helps create more robust and maintainable systems by formalizing the rules of state transitions and preventing invalid state changes through the type system.