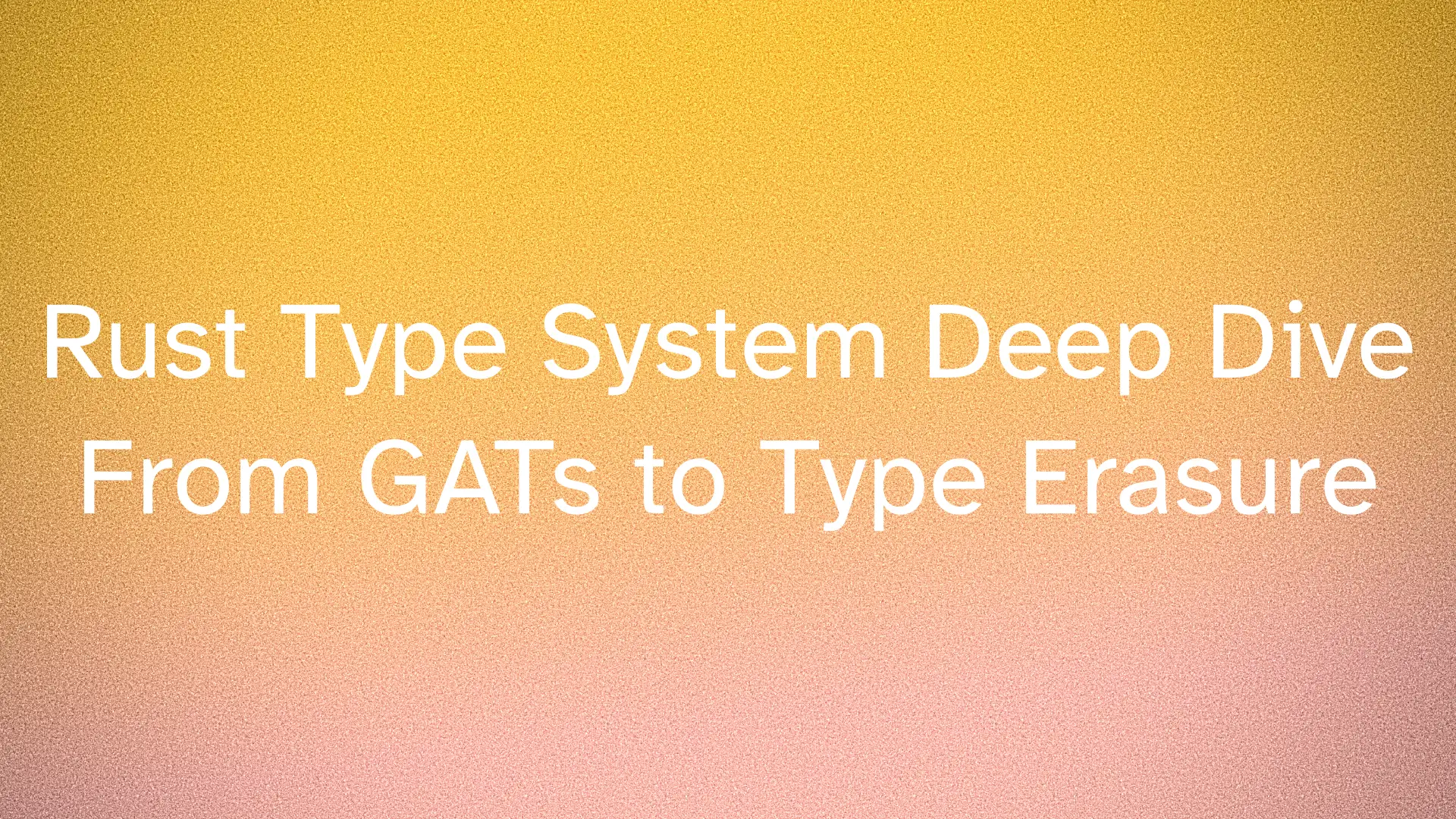
Rust Type System Deep Dive From GATs to Type Erasure
- Introduction
- Generic Associated Types (GATs)
- Advanced Lifetime Management
- Phantom Types
- The Typeclass Pattern
- Zero-Sized Types (ZSTs)
- Type Erasure Patterns
- Conclusion
Introduction
Have you ever stared at a complex Rust error involving lifetimes and wondered if there’s a way to bend the type system to your will? Or perhaps you’ve built a library that relied on runtime checks, wishing you could somehow encode those constraints at compile time?
You’re not alone.
Rust’s type system is like an iceberg — most developers only interact with the visible 10% floating above the water. But beneath the surface lies a world of powerful abstractions waiting to be harnessed.
In this post, we’ll dive deep beneath the surface to explore five advanced type system features that can transform how you design Rust code:
- Generic Associated Types (GATs) — The feature that took 6+ years to stabilize, enabling entirely new categories of APIs
- Advanced Lifetime Management — Techniques to express complex relationships between references
- Phantom Types — Using “ghost” type parameters to encode states with zero runtime cost
- Typeclass Patterns — Bringing functional programming’s power to Rust’s trait system
- Zero-Sized Types (ZSTs) — Types that exist only at compile time but provide powerful guarantees
- Type Erasure Techniques — Methods to hide implementation details while preserving behavior
Why should you care about these advanced patterns? Because they represent the difference between:
- Runtime checks vs. compile-time guarantees
- Documentation comments vs. compile errors for incorrect usage
- Hoping users read your docs vs. ensuring they can’t misuse your API
Let’s begin our journey into the depths of Rust’s type system. By the end, you’ll have new tools to craft APIs that are both more expressive and more robust.
Generic Associated Types (GATs)
The Long Road to Stabilization
“Is it possible to define a trait where the associated type depends on the self lifetime?”
This seemingly innocent question, asked over and over in the Rust community for years, pointed to a critical gap in Rust’s type system. Generic Associated Types (GATs) represent one of Rust’s most anticipated features, finally stabilized in Rust 1.65 after more than six years in development.
The journey to stabilization wasn’t just a matter of implementation — it involved fundamental questions about Rust’s type system design. You might wonder: what kind of feature takes more than half a decade to implement? The answer: one that touches the very core of how generics, traits, and lifetimes interact.
What Are GATs?
Before GATs, you found yourself trapped in situations like this:
trait Container {
type Item;
fn get(&self) -> Option<&Self::Item>;
}
This seems reasonable until you try implementing it for a type like Vec<T>
:
impl<T> Container for Vec<T> {
type Item = T;
fn get(&self) -> Option<&Self::Item> {
// Wait... this doesn't quite work!
// The lifetime of the returned reference comes from `&self`
// but our associated type doesn't know about that lifetime
self.first()
}
}
With GATs, we can make associated types aware of lifetimes:
trait Container {
type Item<'a>
where
Self: 'a;
fn get<'a>(&'a self) -> Option<Self::Item<'a>>;
}
impl<T> Container for Vec<T> {
type Item<'a>
= &'a T
where
Self: 'a;
fn get<'a>(&'a self) -> Option<Self::Item<'a>> {
self.first()
}
}
This seemingly small addition unlocks entirely new categories of APIs that were previously impossible or required unsafe workarounds.
🔑 Key Takeaway: GATs let you create associated types that can reference the lifetime of
&self
, allowing for APIs that were previously impossible to express safely.
Real-world Example: A Collection Iterator Factory
Let’s see how GATs enable elegant APIs for creating iterators with different lifetimes:
trait CollectionFactory {
type Collection<'a> where Self: 'a;
type Iterator<'a>: Iterator where Self: 'a;
fn create_collection<'a>(&'a self) -> Self::Collection<'a>;
fn iter<'a>(&'a self) -> Self::Iterator<'a>;
}
struct VecFactory<T>(Vec<T>);
impl<T: Clone> CollectionFactory for VecFactory<T> {
type Collection<'a> = Vec<T> where T: 'a;
type Iterator<'a> = std::slice::Iter<'a, T> where T: 'a;
fn create_collection<'a>(&'a self) -> Vec<T> {
self.0.clone()
}
fn iter<'a>(&'a self) -> std::slice::Iter<'a, T> {
self.0.iter()
}
}
Before GATs, this pattern would have required boxing, unsafe code, or simply wouldn’t have been possible. Now it’s type-safe and zero-cost.
Think of GATs as the tool that lets you build APIs that adapt to their context — rather than forcing users to adapt to your API.
Advanced Lifetime Management
Lifetimes in Rust are like the air we breathe — essential, ever-present, but often invisible until something goes wrong. Advanced lifetime management gives you the tools to work with this invisible force.
Higher-Rank Trait Bounds (HRTBs)
You’ve likely encountered this cryptic syntax before, maybe in compiler errors:
for<'a> T: Trait<'a>
This strange-looking for<'a>
is the gateway to higher-rank trait bounds. But what does it actually mean?
Imagine you’re writing an API to parse strings:
trait Parser {
fn parse(&self, input: &str) -> Output;
}
But wait — the input’s lifetime is tied to the function call, not the trait definition. Traditional generics can’t express this relationship properly. Instead, we need HRTBs:
trait Parser {
fn parse<F, O>(&self, f: F) -> O
where
F: for<'a> FnOnce(&'a str) -> O;
}
Now we can implement the Parser
trait for our SimpleParser
:
struct SimpleParser;
impl Parser for SimpleParser {
fn parse<F, O>(&self, f: F) -> O
where
F: for<'a> FnOnce(&'a str) -> O,
{
let data = "sample data";
f(data)
}
}
The for<'a>
syntax is a universal quantification over lifetimes, meaning “for all possible lifetimes ‘a”. It’s saying that F
must be able to handle a string slice with any lifetime, not just a specific one determined in advance.
🔑 Key Takeaway: Higher-rank trait bounds let you express relationships between lifetimes that can’t be captured with simple lifetime parameters, enabling more flexible APIs.
Lifetime Variance and ‘Static
Imagine you’re designing an authentication system:
struct AdminToken<'a>(&'a str);
struct UserToken<'a>(&'a str);
fn check_admin_access(token: AdminToken) -> bool {
// Verification logic
true
}
A critical question arises: Could someone pass a UserToken
where an AdminToken
is required? The answer depends on variance.
Variance determines when one type can be substituted for another based on their lifetime relationships:
- Covariant: If
'a
outlives'b
, thenT<'a>
can be used whereT<'b>
is expected (most common) - Contravariant: The opposite relationship
- Invariant: No substitution is allowed (critical for security)
For example, &'a T
is covariant over 'a
, meaning you can use a longer-lived reference where a shorter-lived one is expected:
fn needs_short_lived<'a, 'b: 'a>(data: &'a u32) {
// Some code
}
fn provide_longer_lived<'long>(long_lived: &'long u32) {
needs_short_lived(long_lived); // This works because of covariance
}
Understanding these relationships becomes essential when designing APIs that deal with sensitive resources or complex lifetime interactions.
Phantom Types
Have you ever wished you could distinguish between two values of the same type but with different meanings? Consider these examples:
// These are all just strings, but they have very different meanings!
let user_id = "usr_123456";
let order_id = "ord_789012";
let coupon_code = "SAVE20";
Nothing prevents you from accidentally mixing them up. This is where phantom types come in — they let you create type-level distinctions without runtime cost.
Phantom types are type parameters that don’t appear in the data they parameterize:
use std::marker::PhantomData;
struct Token<State> {
value: String,
_state: PhantomData<State>,
}
The PhantomData<State>
field takes no space at runtime, but it creates a distinct type at compile time.
🔑 Key Takeaway: Phantom types allow you to encode additional information in the type system without adding any runtime overhead, creating distinctions that exist purely at compile time.
State Machines at Compile Time
One of the most powerful applications of phantom types is encoding state machines directly in the type system:
// States (empty structs)
struct Unvalidated;
struct Validated;
// Validation error type
enum ValidationError {
TooShort,
InvalidFormat,
}
impl Token<Unvalidated> {
fn new(value: String) -> Self {
Token {
value,
_state: PhantomData,
}
}
fn validate(self) -> Result<Token<Validated>, ValidationError> {
// Perform validation
if self.value.len() > 3 {
Ok(Token {
value: self.value,
_state: PhantomData,
})
} else {
Err(ValidationError::TooShort)
}
}
}
impl Token<Validated> {
fn use_validated(&self) -> &str {
// Only callable on validated tokens
&self.value
}
}
This pattern ensures that use_validated()
can only be called on tokens that have passed validation, with the guarantee enforced at compile time.
Type-Level Validation
Phantom types can encode domain-specific rules at the type level, essentially moving validation from runtime to compile time:
struct UserId<Validated>(String, PhantomData<Validated>);
struct EmailAddress<Validated>(String, PhantomData<Validated>);
struct Unverified;
struct Verified;
trait Validator<T> {
type Error;
fn validate(value: String) -> Result<T, Self::Error>;
}
struct UserIdValidator;
impl Validator<UserId<Verified>> for UserIdValidator {
type Error = String;
fn validate(value: String) -> Result<UserId<Verified>, Self::Error> {
if value.len() >= 3 && value.chars().all(|c| c.is_alphanumeric()) {
Ok(UserId(value, PhantomData))
} else {
Err("Invalid user ID".to_string())
}
}
}
// Now functions can require verified types
fn register_user(id: UserId<Verified>, email: EmailAddress<Verified>) {
// We know both ID and email have been validated
}
This approach creates a “validation firewall” — once data passes through validation, its type guarantees it’s valid throughout the rest of your program.
The Typeclass Pattern
What if you could define behavior for types you don’t control?
Haskell programmers have long enjoyed typeclasses, a powerful mechanism for defining interfaces that types can implement. Rust’s trait system offers similar capabilities, but we can go further to implement true typeclass-style programming.
What Are Typeclasses?
Imagine you’re building a serialization library and want to support many different formats. Without typeclasses, you’d need to:
- Create a trait
- Implement it for every type you own
- Hope other library authors implement it for their types
- Resort to newtype wrappers for types you don’t control
In functional languages like Haskell, typeclasses solve this elegantly by allowing you to define behavior for any type, even ones you didn’t create. Rust’s trait system gives us similar power through “orphan implementations” (with some restrictions).
The key components of typeclass patterns in Rust are:
- Traits as interfaces
- Trait implementations for existing types (including foreign types)
- Associated types or type parameters for related types
- Trait bounds to express constraints
🔑 Key Takeaway: Typeclasses let you add behavior to types after they’re defined, enabling powerful generic programming.
From Monoids to Semigroups
Let’s dive into some algebraic abstractions to see typeclasses in action:
trait Semigroup {
fn combine(&self, other: &Self) -> Self;
}
trait Monoid: Semigroup + Clone {
fn empty() -> Self;
}
// Implementing for built-in types
impl Semigroup for String {
fn combine(&self, other: &Self) -> Self {
let mut result = self.clone();
result.push_str(other);
result
}
}
impl Monoid for String {
fn empty() -> Self {
String::new()
}
}
// Product and Sum types for numbers
#[derive(Clone)]
struct Product<T>(T);
#[derive(Clone)]
struct Sum<T>(T);
impl<T: Clone + std::ops::Mul<Output = T>> Semigroup for Product<T> {
fn combine(&self, other: &Self) -> Self {
Product(self.0.clone() * other.0.clone())
}
}
impl<T: Clone + std::ops::Mul<Output = T> + From<u8>> Monoid for Product<T> {
fn empty() -> Self {
Product(T::from(1))
}
}
impl<T: Clone + std::ops::Add<Output = T>> Semigroup for Sum<T> {
fn combine(&self, other: &Self) -> Self {
Sum(self.0.clone() + other.0.clone())
}
}
impl<T: Clone + std::ops::Add<Output = T> + From<u8>> Monoid for Sum<T> {
fn empty() -> Self {
Sum(T::from(0))
}
}
You might be thinking: “That looks like a lot of boilerplate just to add strings or multiply numbers.” But the magic happens when we build generic algorithms that work with any type that implements our traits.
Leveraging Typeclasses for Generic Algorithms
Once we have these abstractions, we can write algorithms that work with any Monoid, regardless of the actual data type:
fn combine_all<M: Monoid + Clone>(values: &[M]) -> M {
values.iter().fold(M::empty(), |acc, x| acc.combine(x))
}
// Usage
let strings = vec![String::from("Hello, "), String::from("typeclasses "), String::from("in Rust!")];
let result = combine_all(&strings);
// "Hello, typeclasses in Rust!"
let numbers = vec![Sum(1), Sum(2), Sum(3), Sum(4)];
let sum_result = combine_all(&numbers);
// Sum(10)
let products = vec![Product(2), Product(3), Product(4)];
let product_result = combine_all(&products);
// Product(24)
With just a few lines of code, we’ve created a function that can concatenate strings, sum numbers, multiply numbers, or work with any other type that follows the Monoid abstraction. This is the power of typeclass-based programming!
Zero-Sized Types (ZSTs)
Zero-sized types (ZSTs) are types that occupy no memory at runtime but carry semantic meaning at compile time. They’re a powerful tool for type-level programming without runtime overhead.
What Are Zero-Sized Types?
A ZST is any type that requires 0 bytes of storage. Common examples include:
- Empty structs:
struct Marker;
- Empty enums:
enum Void {}
- PhantomData:
PhantomData<T>
- Unit type:
()
Despite taking no space, ZSTs provide valuable type information to the compiler.
Marker Types
One common use of ZSTs is as marker types to implement compile-time flags:
// Markers for access levels
struct ReadOnly;
struct ReadWrite;
struct Database<Access> {
connection_string: String,
_marker: PhantomData<Access>,
}
impl<Access> Database<Access> {
fn query(&self, query: &str) -> Vec<String> {
// Common query logic
vec![format!("Result of {}", query)]
}
}
impl Database<ReadWrite> {
fn execute(&self, command: &str) -> Result<(), String> {
// Only available in read-write mode
Ok(())
}
}
// Usage
let read_only_db = Database::<ReadOnly> {
connection_string: "sql://readonly".to_string(),
_marker: PhantomData,
};
let read_write_db = Database::<ReadWrite> {
connection_string: "sql://admin".to_string(),
_marker: PhantomData,
};
read_only_db.query("SELECT * FROM users");
// read_only_db.execute("DROP TABLE users"); // Won't compile!
read_write_db.execute("INSERT INTO users VALUES (...)"); // Works
Type-Level State Machines with ZSTs
ZSTs excel at encoding state machines where state transitions happen at compile time:
// States
struct Draft;
struct Published;
struct Archived;
// Post with type-level state
struct Post<State> {
content: String,
_state: PhantomData<State>,
}
// Operations available on Draft posts
impl Post<Draft> {
fn new(content: String) -> Self {
Post {
content,
_state: PhantomData,
}
}
fn edit(&mut self, content: String) {
self.content = content;
}
fn publish(self) -> Post<Published> {
Post {
content: self.content,
_state: PhantomData,
}
}
}
// Operations available on Published posts
impl Post<Published> {
fn get_views(&self) -> u64 {
42 // Placeholder
}
fn archive(self) -> Post<Archived> {
Post {
content: self.content,
_state: PhantomData,
}
}
}
// Operations available on Archived posts
impl Post<Archived> {
fn restore(self) -> Post<Draft> {
Post {
content: self.content,
_state: PhantomData,
}
}
}
Type-Level Integers and Dimensional Analysis
With const generics, we can use ZSTs to encode types with numeric properties:
// Type-level integers with const generics
struct Length<const METERS: i32, const CENTIMETERS: i32>;
// Type-level representation of physical quantities
impl<const M: i32, const CM: i32> Length<M, CM> {
// A const function to calculate total centimeters (for demonstration)
const fn total_cm() -> i32 {
M * 100 + CM
}
}
// Type-safe addition using type conversion rather than type-level arithmetic
fn add<const M1: i32, const CM1: i32, const M2: i32, const CM2: i32>(
_: Length<M1, CM1>,
_: Length<M2, CM2>,
) -> Length<3, 120> {
// Using fixed return type for the example
// In a real implementation, we would define constant expressions and
// use const generics with a more flexible type, but that gets complex
Length
}
// Usage
let a = Length::<1, 50> {};
let b = Length::<2, 70> {};
let c = add(a, b); // Type is Length<3, 120>
Optimizations with ZSTs
Because ZSTs take no space, the compiler can optimize away operations with them while preserving their type-level semantics:
- Collections of ZSTs take no space
- Functions returning ZSTs are optimized to simple jumps
- Fields of type ZST don’t increase struct size
This makes ZSTs perfect for:
- Type-level programming
- Differentiating between identical data layouts with different semantics
- Building extensible APIs with marker traits
Type Erasure Patterns
Type erasure is a powerful technique for hiding concrete types behind abstract interfaces while maintaining type safety. In Rust, there are several ways to implement type erasure, each with different trade-offs.
Understanding Type Erasure
Type erasure refers to the process of “erasing” or hiding concrete type information while preserving the necessary behavior. This allows for:
- Handling multiple types uniformly
- Creating heterogeneous collections
- Simplifying complex generic interfaces
- Providing abstraction boundaries in APIs
Dynamic Trait Objects
The most common form of type erasure in Rust uses trait objects with dynamic dispatch:
trait Drawable {
fn draw(&self);
fn bounding_box(&self) -> BoundingBox;
}
struct BoundingBox {
x: f32,
y: f32,
width: f32,
height: f32,
}
struct Rectangle {
x: f32,
y: f32,
width: f32,
height: f32,
}
impl Drawable for Rectangle {
fn draw(&self) {
// Draw the rectangle
}
fn bounding_box(&self) -> BoundingBox {
BoundingBox {
x: self.x,
y: self.y,
width: self.width,
height: self.height,
}
}
}
struct Circle {
x: f32,
y: f32,
radius: f32,
}
impl Drawable for Circle {
fn draw(&self) {
// Draw the circle
}
fn bounding_box(&self) -> BoundingBox {
BoundingBox {
x: self.x - self.radius,
y: self.y - self.radius,
width: self.radius * 2.0,
height: self.radius * 2.0,
}
}
}
Now we can create a Canvas
that can hold different types of Drawable
objects:
struct Canvas {
// A collection of drawable objects with different concrete types
elements: Vec<Box<dyn Drawable>>,
}
impl Canvas {
fn new() -> Self {
Canvas {
elements: Vec::new(),
}
}
fn add_element<T: Drawable + 'static>(&mut self, element: T) {
self.elements.push(Box::new(element));
}
fn draw_all(&self) {
for element in &self.elements {
element.draw();
}
}
}
This approach uses runtime polymorphism (vtables) to call the correct implementation. The concrete type is erased, but at the cost of dynamic dispatch and heap allocation.
The Object-Safe Trait Pattern
Creating object-safe traits requires careful design:
// Non-object-safe trait with generic methods
trait NonObjectSafe {
fn process<T>(&self, value: T);
}
// Object-safe wrapper
trait ObjectSafe {
fn process_i32(&self, value: i32);
fn process_string(&self, value: String);
// Add concrete methods for each type you need
}
// Bridge implementation
impl<T: NonObjectSafe> ObjectSafe for T {
fn process_i32(&self, value: i32) {
self.process(value);
}
fn process_string(&self, value: String) {
self.process(value);
}
}
This pattern allows you to create trait objects from traits that would otherwise not be object-safe, at the cost of some flexibility.
Building Heterogeneous Collections
Type erasure is particularly useful for creating collections of different types:
trait Message {
fn process(&self);
}
// Type-erased message holder
struct AnyMessage {
inner: Box<dyn Message>,
}
// Specific message types
struct TextMessage(String);
struct BinaryMessage(Vec<u8>);
impl Message for TextMessage {
fn process(&self) {
println!("Processing text: {}", self.0);
}
}
impl Message for BinaryMessage {
fn process(&self) {
println!("Processing binary data of size: {}", self.0.len());
}
}
// Usage
fn main() {
let messages: Vec<AnyMessage> = vec![
AnyMessage { inner: Box::new(TextMessage("Hello".to_string())) },
AnyMessage { inner: Box::new(BinaryMessage(vec![1, 2, 3, 4])) },
];
for msg in messages {
msg.inner.process();
}
}
For performance-critical code, you might use an enum-based approach instead:
enum MessageKind {
Text(String),
Binary(Vec<u8>),
}
impl MessageKind {
fn process(&self) {
match self {
MessageKind::Text(text) => {
println!("Processing text: {}", text);
}
MessageKind::Binary(data) => {
println!("Processing binary data of size: {}", data.len());
}
}
}
}
This approach avoids the dynamic dispatch overhead but requires enumerating all possible types upfront.
Conclusion
We’ve journeyed deep into Rust’s type system, exploring powerful Rust features. Let’s recap what we’ve discovered:
- Generic Associated Types (GATs) — The feature years in the making that lets you create associated types that depend on lifetimes, enabling entirely new categories of safe APIs.
- Advanced Lifetime Management — Techniques like higher-rank trait bounds and lifetime variance that give you fine-grained control over how references relate to each other.
- Phantom Types — “Ghost” type parameters that take no space at runtime but create powerful type distinctions, perfect for encoding state machines and validation requirements.
- Typeclass Patterns — Functional programming techniques brought to Rust, enabling highly generic code that works across different types through trait abstraction.
- Zero-Sized Types (ZSTs) — Types that exist only at compile time but provide powerful guarantees with zero runtime cost, from marker traits to dimensional analysis.
- Type Erasure Techniques — Methods to hide implementation details while preserving behavior, essential for creating clean API boundaries and heterogeneous collections.
So what should you do with this knowledge?
The next time you find yourself writing:
- Runtime checks that could be compile-time guarantees
- Documentation about how API functions must be called in a certain order
- Warning comments about not mixing up similar-looking values
- Complex validation logic scattered throughout your codebase
…consider whether one of these type system features could solve your problem more elegantly.
The beauty of Rust’s type system is that it turns the compiler into your ally. Instead of fighting with it, you can teach it to catch your domain-specific errors before your code even runs.